OTT Express SDK
OTT Express
The best in Native and React Native component library and SDKs for robust and rapid app development.
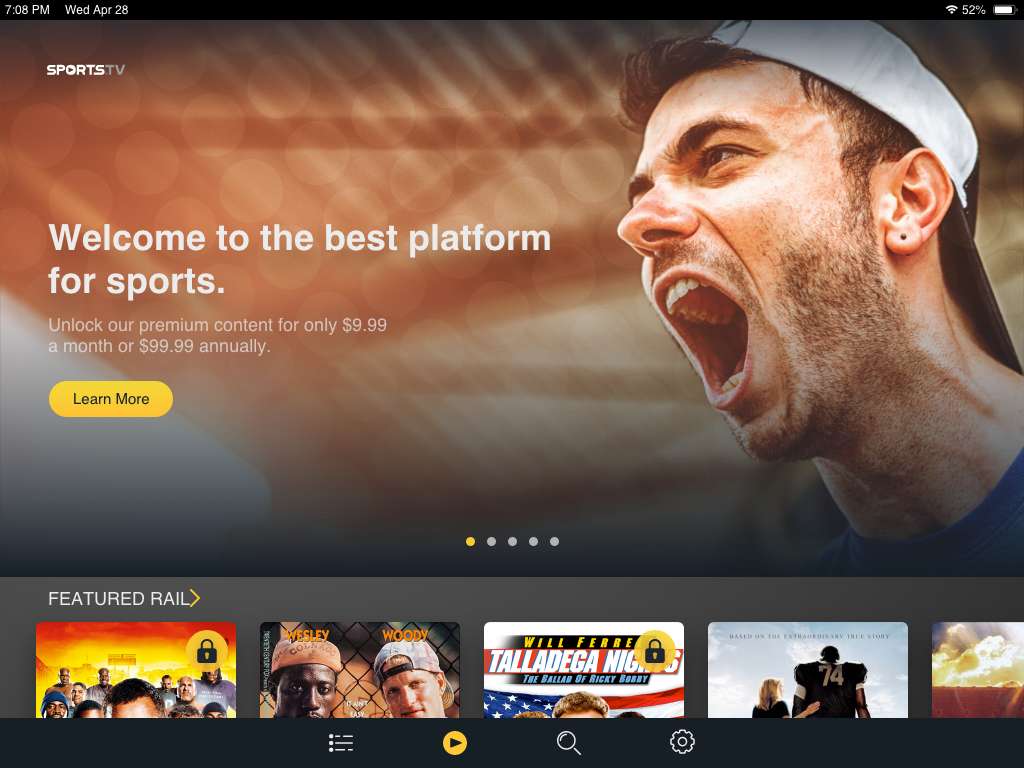
Homepage
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
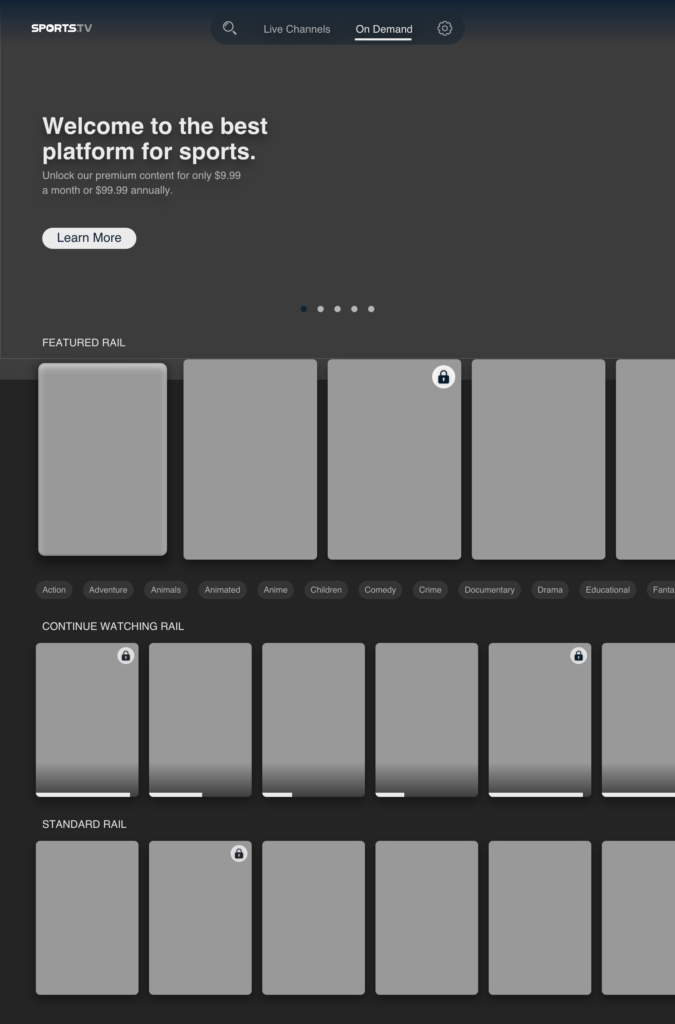
Sample Code:
============= Home Screen ===================================
class DSHomeScreen extends SPLTHomeScreen {
carouselItemClick = (spltContent: SPLTContent) => {};
railItemClick = (spltContent: SPLTContent) => {
this.props.navigation.navigate('DSPDP', {
spltContent,
type: typeof spltContent,
});
};
}
const mapStateToProps = state => ({
error: state.spltAppLoadReducer.error,
spltContents: state.spltAppLoadReducer.spltCategories,
theme: theme,
style: style,
config: config,
});
export default connect(mapStateToProps, {spltAppLoadActions})(DSHomeScreen);
Dynamic On Demand Rail Pages
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
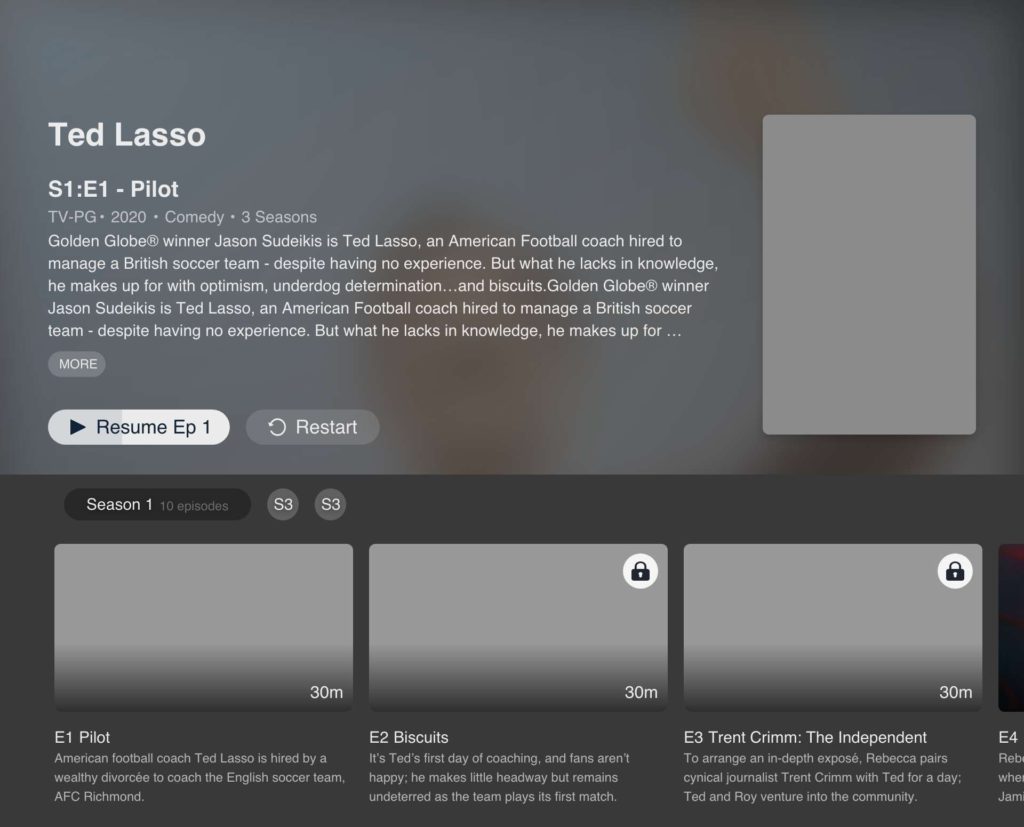
============= PDP Screen ===================================
class DSPDPScreen extends SPLTPDPScreen {
componentDidMount() {
this.props.spltPDPActionsData(this.props.route.params?.spltContent);
}
function mapDispatchToProps(dispatch: any) {
return {
spltPDPActionsData: (spltContent: SPLTContent) =>
dispatch(spltPDPActions(spltContent)),
clearChannelActions: () => dispatch(clearChannelActions()),
};
}
function mapStateToProps(state) {
let recommendationSpltCategory = state.spltRecommendationReducer
.spltCategory as SPLTRecommendationCategory;
recommendationSpltCategory.style.cellImageStyle.aspectRatio = 2.0 / 3.0;
if (DeviceInfo.isTablet()) {
recommendationSpltCategory.style.cellStyle.widthPercentage = 17;
recommendationSpltCategory.style.railStyle.itemSpacingPercentage = 5;
} else if (Platform.isTV) {
recommendationSpltCategory.style.cellStyle.widthPercentage = 17;
} else {
recommendationSpltCategory.style.cellStyle.widthPercentage = 35;
}
return {
error: state.spltPDPReducer?.error,
spltChannel: state.spltPDPReducer.spltChannel,
recommendationError:state.spltRecommendationReducer.error,
recommendationSpltCategory: recommendationSpltCategory,
ratioData: state.spltPDPReducer?.ratioData ? state.spltPDPReducer?.ratioData : 0.6667,
theme,
style,
config,
};
}
export default connect(mapStateToProps, mapDispatchToProps)(DSPDPScreen);
Program Guide Schedule Pages
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
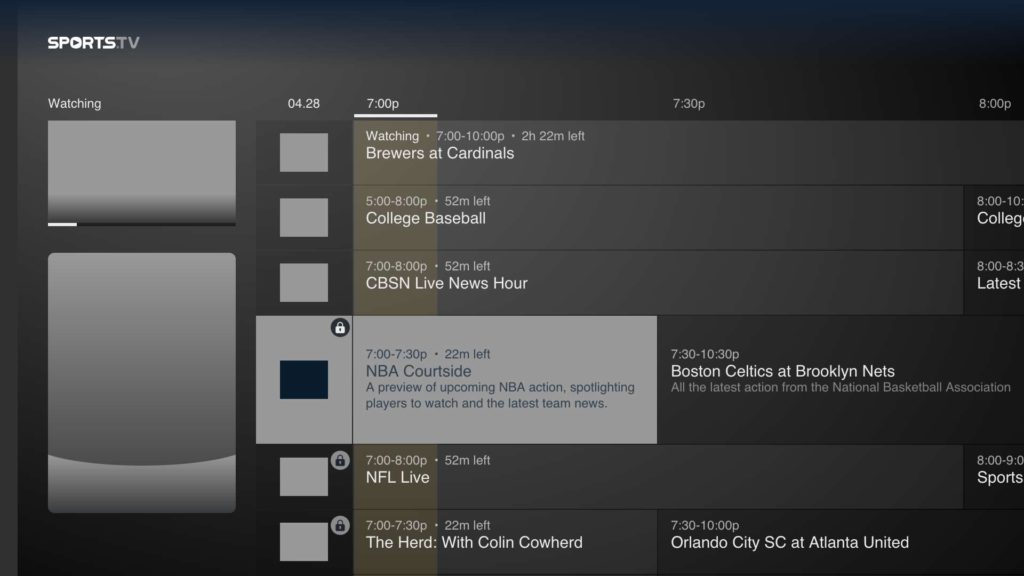
============= EPG Screen ===================================
class DSEPGScreen extends SPLTEPGScreen {
fromDay: number = 0;
}
function mapStateToProps(state) {
return {
EPGConfig: EPGStyle.EPGConfig,
theme: EPGStyle.themes,
style: EPGStyle.styles,
promo: state.spltPromoReducer.spltPromoChannels,
promoImage: state.spltPromoReducer.spltPromoChannels.spotlight_poster,
marketingURL: state.spltPromoReducer.spltPromoChannels.strSlug,
PLAYER_IMAGE_SAMPLE: EPGDrawable.PLAYER_IMAGE_SAMPLE,
PLAY_ICON: IconsDrawable.PLAY_ICON,
BOUNCING_BALL: bouncingBall,
SPLASH_BACKGROUND: splashDrawable.splash_bg,
error: state.spltEPGReducer.error,
spltEPGChannels: state.spltEPGReducer.spltEPGChannels,
fromDay: state.spltEPGReducer.fromDay,
spltVideo: state.spltVideoReducer.spltVideo,
isLoading: state.spltEPGReducer.isLoading,
};
}
const mapDispatchToProps = dispatch => {
return {
spltVideoActionsData: (spltVideo: SPLTVideo) =>
dispatch(spltVideoActions(spltVideo)),
spltEPGActions: (
from: number,
size: number,
fromDay: number,
) => dispatch(spltEPGActions(from, size, isLowerDevice, fromDay)),
spltPromoActions: () => dispatch(spltPromoActions()),
};
};
export default connect(mapStateToProps, mapDispatchToProps)(DSEPGScreen);
============= Player Screen ===================================
class DSPlayerScreen extends SPLTPlayerScreen {
componentDidMount() {
super.componentDidMount();
this.setState({spltChannel: this.props.route.params.spltChannel});
}
}
function mapStateToProps(state) {
return {
theme: player.themes,
style: player.styles,
error: state.spltEPGReducer.error,
spltEPGChannel: state.spltEPGReducer.spltEPGChannel,
};
}
const mapDispatchToProps = dispatch => ({});
export default connect(mapStateToProps, mapDispatchToProps)(DSPlayerScreen);
Elegant Player Controls
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
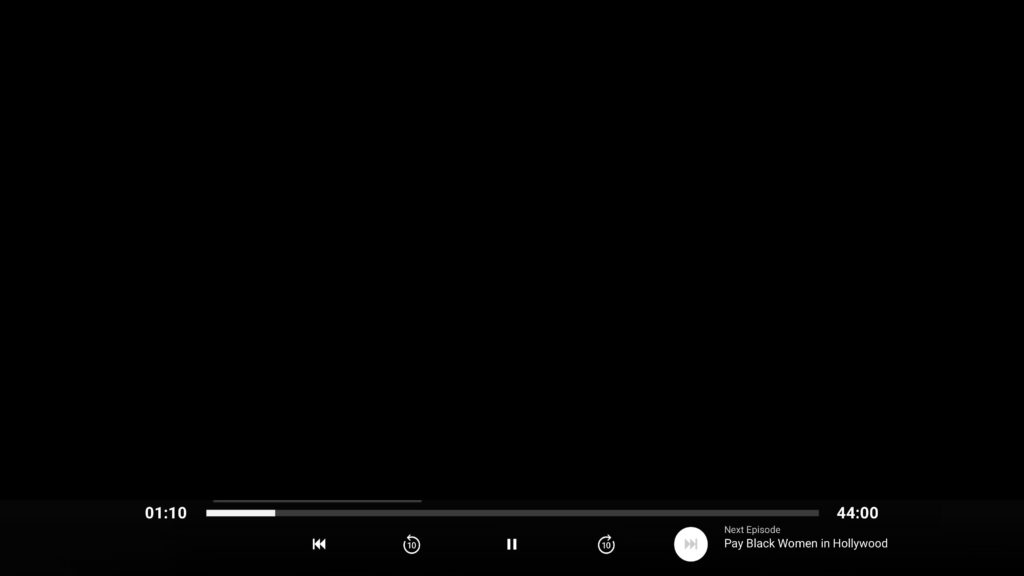
Subscription Plans
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
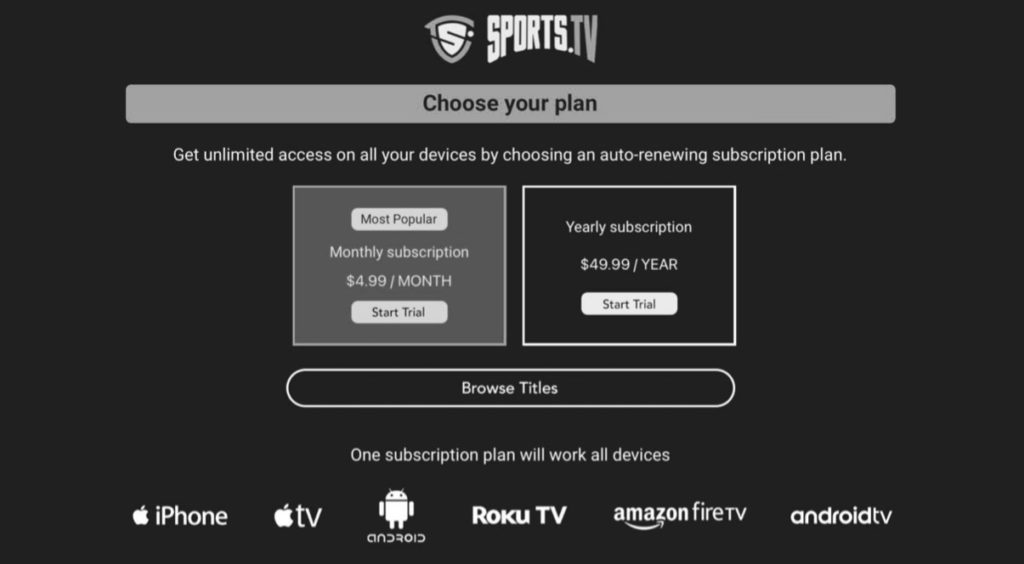
============= Subscription Screen ===================================
class DSProductsScreens extends SPLTProductsScreen {
componentDidMount() {
if (this.props.validationType) {
validationType = this.props.validationType
}
if (this.props.channelId) {
channelId = this.props.channelId
}
this.props.spltProductActions(channelId);
}
onClickSubscription = (items: SPLTProduct) => {
};
}
function mapStateToProps(state) {
const spltUserProduct = state.spltUserProductReducer.spltUserProduct;
return {
error: state.spltProductReducer.error,
isLoading: state.spltProductReducer.isLoading,
spltProduct: spltProduct,
theme: theme,
style: style,
};
}
const mapDispatchToProps = dispatch => ({
spltProductActions: channelId => dispatch(spltProductAction(channelId)),
});
export default connect(mapStateToProps, mapDispatchToProps)(DSProductsScreens);
DRM
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
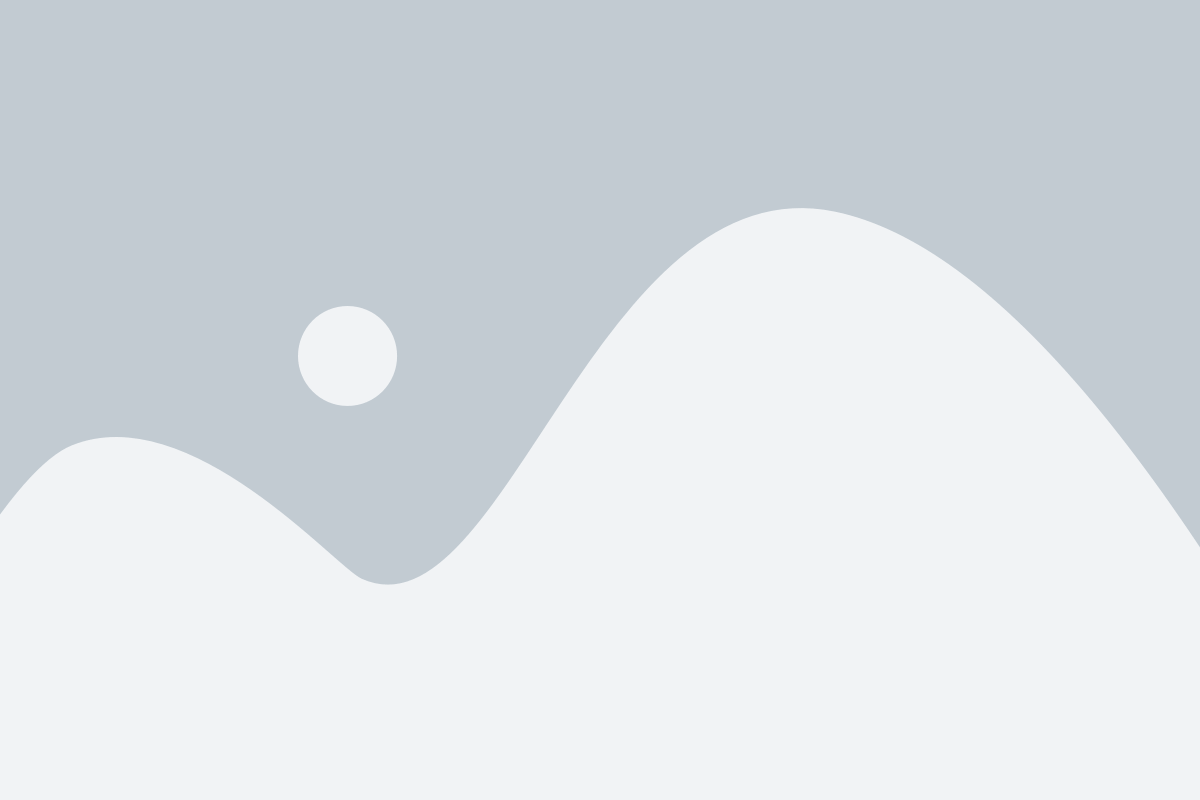
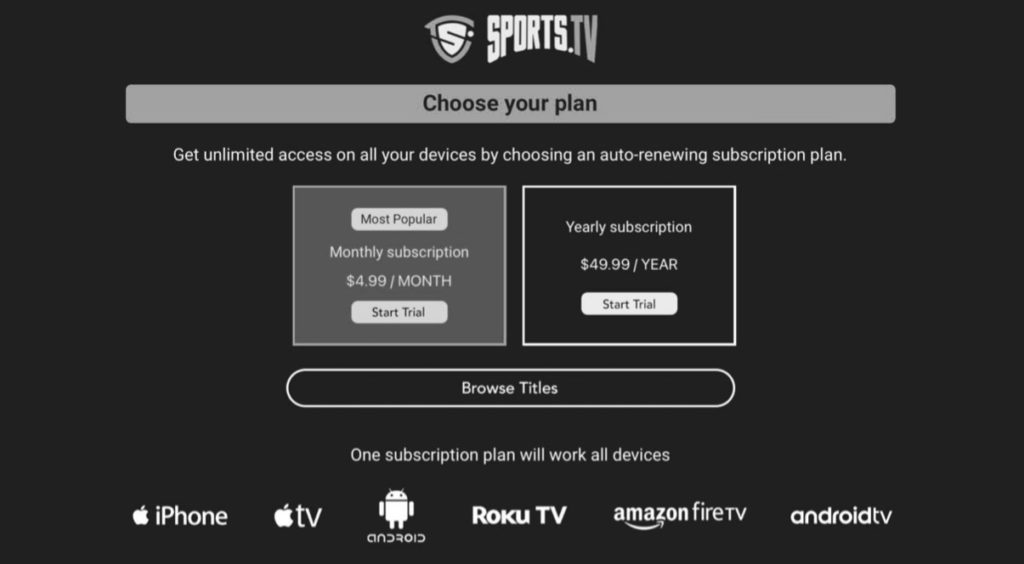
Adobe Primetime
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
============= Authentication Controller ===================================
For Login,
this.props.navigation.navigate('DSAuthController', {
validationType: ValidationType.LOGIN,
channelId: channel.dspro_id,
onAuthenticationSuccess: () => this.onAuthenticationSuccess(),
onAuthenticationFail: () => this.onAuthenticationFail(),
onAuthenticationSkip: () => this.onAuthenticationSkip(),
});
For Subscription,
this.props.navigation.navigate('DSAuthController', {
validationType: ValidationType.SUBSCRIPTION,
channelId: channel.dspro_id,
onAuthenticationSuccess: () => this.onAuthenticationSuccess(),
onAuthenticationFail: () => this.onAuthenticationFail(),
onAuthenticationSkip: () => this.onAuthenticationSkip(),
});
To purchase Channel
this.props.navigation.navigate('DSAuthController', {
validationType: ValidationType.CHANNEL_UNLOCKED,
channelId: channel.dspro_id,
onAuthenticationSuccess: () => this.onAuthenticationSuccess(),
onAuthenticationFail: () => this.onAuthenticationFail(),
onAuthenticationSkip: () => this.onAuthenticationSkip(),
});
Authentication
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
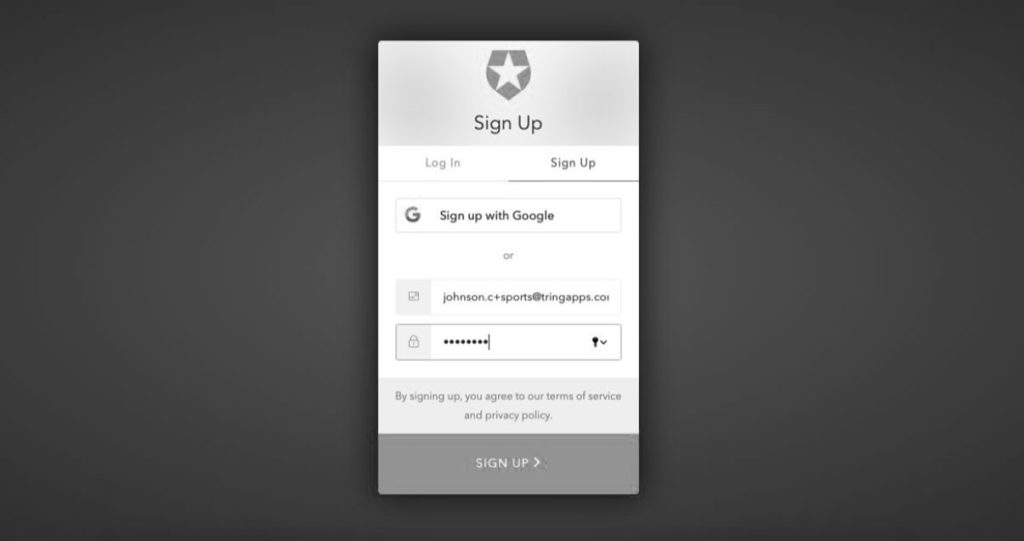
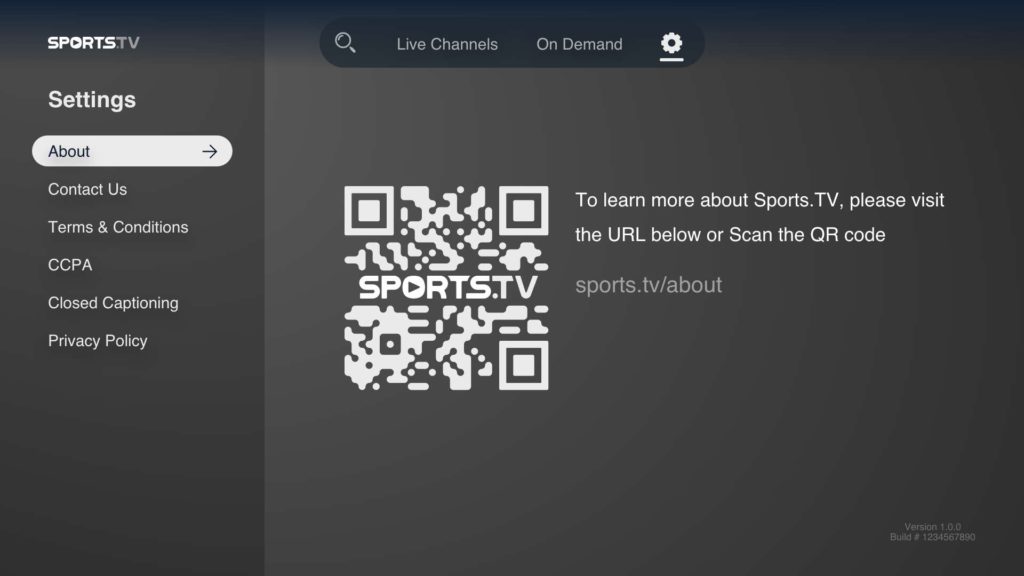
App Settings
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Search
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
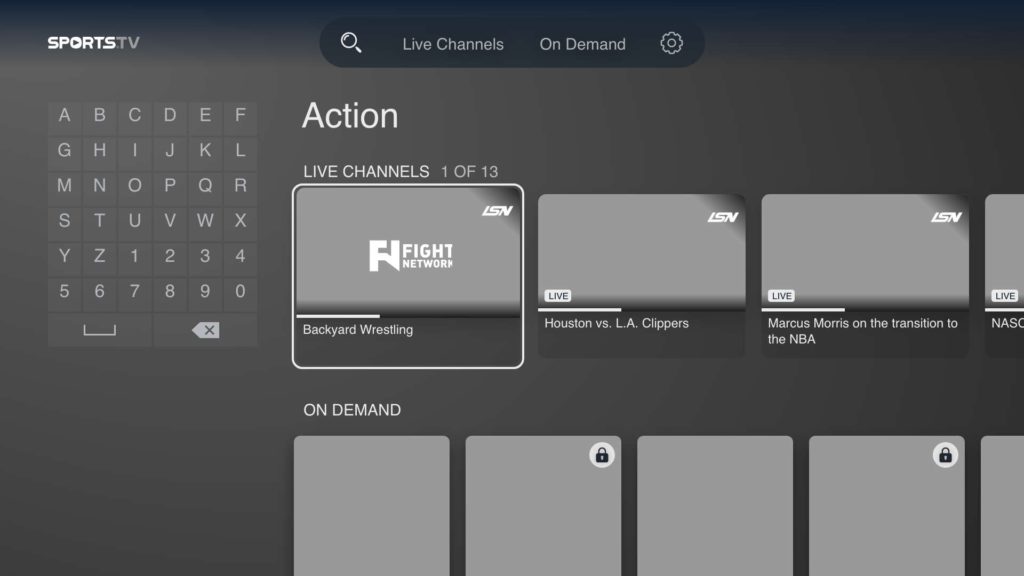
Modular Component Library
OTT Express component library and SDKs for robust and rapid app development.
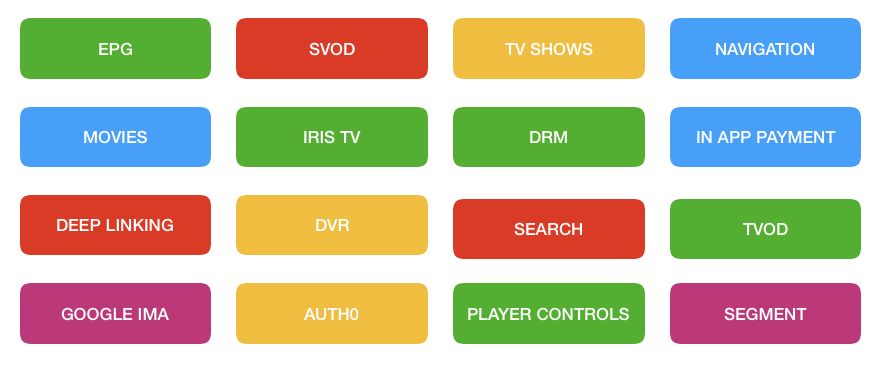
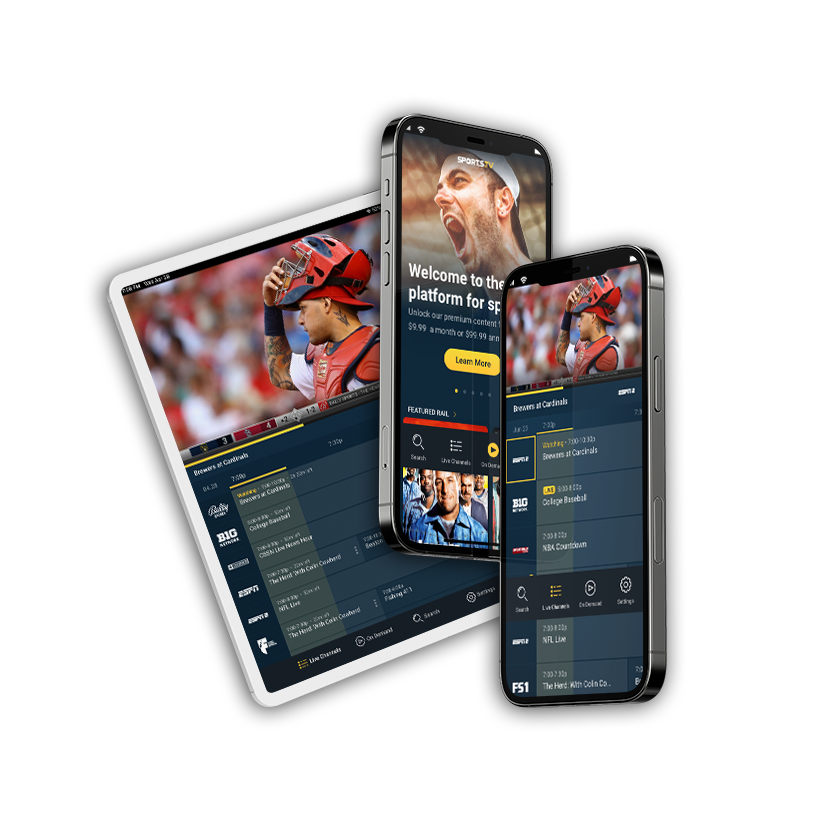
Add Your Heading Text Here
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.